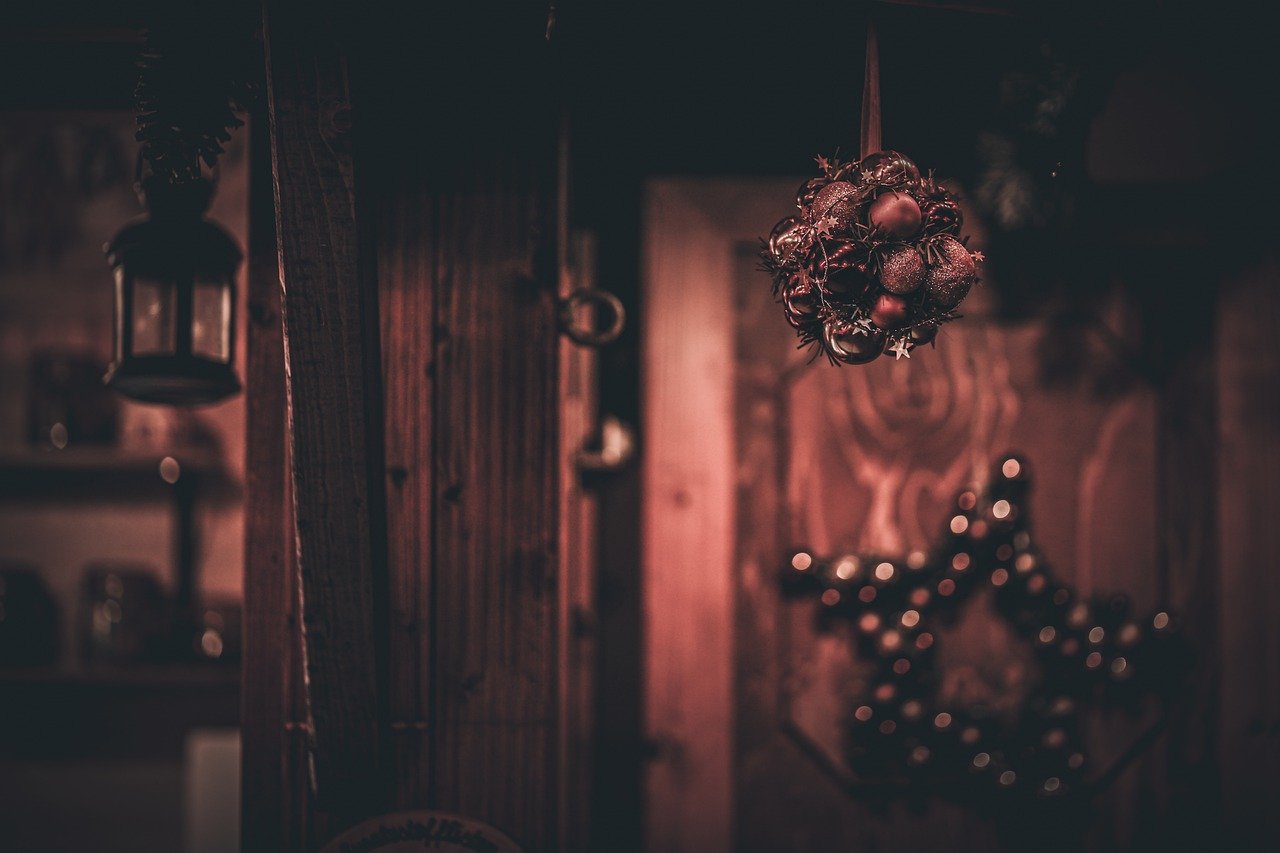
What is DDD (Domain-driven design)?
As a software developer, you want to create high-quality, maintainable code that is well-suited to the needs of your project and your team. To achieve this, it’s important to use a structured approach to software development. One such approach is domain-driven design (DDD), which focuses on the domain, or the problem space, of the application. In this blog post, we’ll discuss what DDD is, and how you can use it to improve the design of your software.
What is domain-driven design?
Domain-driven design (DDD) is a software development approach that focuses on the domain, or the problem space, of the application. DDD emphasizes the importance of understanding the domain, and using that understanding to drive the design of the software.
// Example of a domain model in DDD
class Order {
constructor(id, customer, items) {
this.id = id;
this.customer = customer;
this.items = items;
}
get total() {
let total = 0;
for (const item of this.items) {
total += item.price * item.quantity;
}
return total;
}
}
In the example above, we define a Order class that represents an order in a domain model. The Order class has properties for the order ID, customer, and items, as well as a total method that calculates the total cost of the order. By designing the software in this way, we can better understand the domain and the needs of the application.
How to apply DDD in software development
To apply DDD in your software development, you can follow these steps:
Understand the domain. Start by gaining a deep understanding of the problem space, or domain, of the application. This includes the business domain, the user domain, and the technical domain.
Identify the core domain. Within the domain, identify the core domain, which is the part of the domain that is most important to the business. This is where you should focus your attention, and where you can have the biggest impact.
Model the domain. Create a domain model that represents the key concepts, relationships, and rules of the domain. The domain model should be based on the requirements of the business, and should be expressed in the language of the domain.
Align the code with the model. Use the domain model to drive the design of the software. This means aligning the code with the model, and using the language of the domain in the code.
Iterate and evolve. As you develop the software, iterate and evolve the domain model as needed. This will help to ensure that the model remains accurate and relevant, and that the code continues to align with the model.
// Example of applying DDD in software development
// 1. Understand the domain
const domain = {
business: { … },
user: { … },
technical: { … }
};
// 2. Identify the core domain
const coreDomain = domain.business;
// 3. Model the domain
const domainModel = {
Order: class Order {
constructor(id, customer, items) {
this.id = id;
this.customer = customer;
this.items = items;
}
Copy code
get total() {
let total = 0;
for (const item of this.items) {
total += item.price * item.quantity;
}
return total;
}
}
};
// 4. Align the code with the model
class Order {
constructor(id, customer, items) {
this.id = id;
this.customer = customer;
this.items = items;
}
get total() {
let total = 0;
for (const item of this.items) {
total += item.price * item.quantity;
}
return total;
}
}
// 5. Iterate and evolve
// (continuously refine the domain model and code as needed)
In the example above, we apply the steps of DDD to develop a software application. We start by understanding the domain, and then identify the core domain. We create a domain model that represents the key concepts of the domain. The domain model is then used to drive the design of the software, and the code is aligned with the model. As the software is developed, the domain model is iterated and evolved as needed. By following these steps, you can use DDD to improve the design of your software and create high-quality, maintainable code.
Conclusion:
In this blog post, we’ve discussed what domain-driven design (DDD) is, and how you can apply it to your software development. DDD is a software development approach that focuses on the domain, or problem space, of the application. By understanding the domain and using it to drive the design of the software, you can create high-quality, maintainable code that is well-suited to the needs of your project and your team.