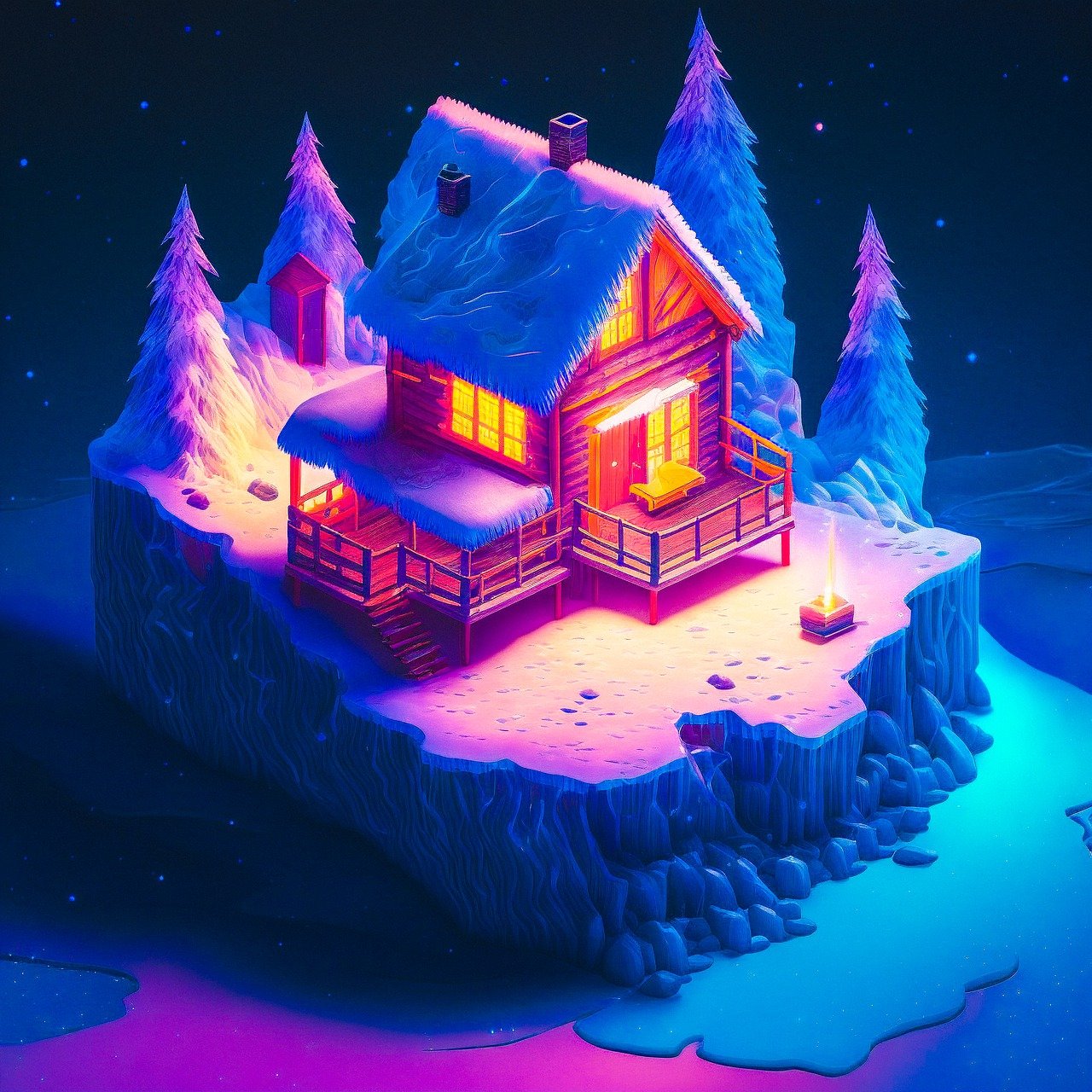
Typescript basics
Introduction to TypeScript
TypeScript is a programming language that is a superset of JavaScript. This means that all valid JavaScript code is also valid TypeScript code. In addition to the features of JavaScript, TypeScript includes optional static typing, classes, and interfaces. This allows developers to write type-safe code and catch errors at compile time, rather than runtime.
Installation and Setup
To use TypeScript, you need to install it on your system. The recommended way to do this is through npm, the package manager for JavaScript. To install TypeScript globally, run the following command:
npm install -g typescript
After installation, you can compile TypeScript code to JavaScript using the tsc
command. For example, to compile a file named main.ts
, you would run the following command:
tsc main.ts
This will create a file named main.js
that contains the JavaScript equivalent of the TypeScript code in main.ts
.
Basic Types
In TypeScript, you can specify the type of a variable when you declare it. This allows the TypeScript compiler to catch type errors and helps you avoid bugs in your code.
Here are some basic types in TypeScript:
number
: This is the type for numeric values.string
: This is the type for string values.boolean
: This is the type for true/false values.any
: This is the type for any value. It is useful when you don’t know the type of a value.
Here is an example of declaring variables with types:
let myNumber: number = 10;
let myString: string = "Hello, world!";
let myBoolean: boolean = true;
let myAny: any = 100;
Classes and Interfaces
In TypeScript, you can define classes and interfaces to create complex types. A class is a blueprint for an object, and an interface is a way to define a contract for the shape of an object.
Here is an example of a class and an interface in TypeScript:
class Point {
x: number;
y: number;
constructor(x: number, y: number) {
this.x = x;
this.y = y;
}
getDistance() {
return Math.sqrt(this.x * this.x + this.y * this.y);
}
}
interface Movable {
move(distance: number): void;
}
class MovablePoint implements Movable {
x: number;
y: number;
constructor(x: number, y: number) {
this.x = x;
this.y = y;
}
move(distance: number) {
this.x += distance;
this.y += distance;
}
}
In the example above, the Point
class has two properties, x
and y
, and a method named getDistance
. The MovablePoint
class extends the Point
class and implements the Movable
interface, which requires it to have a move
method.
Conclusion
TypeScript is a powerful language that offers many