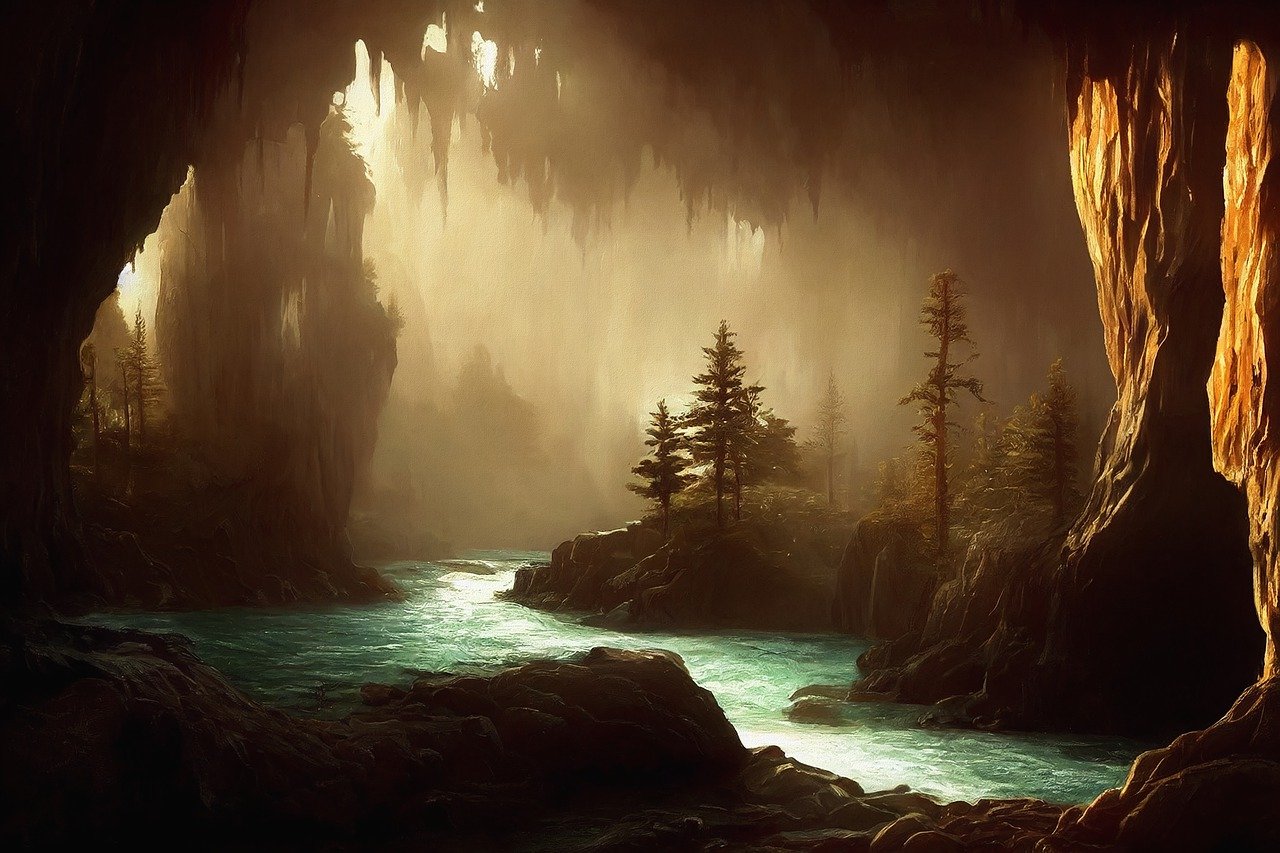
Solid Principals
Software design is an important aspect of the software development process, as it determines the overall structure and organization of the codebase. One of the key principles that software designers follow is the SOLID principles, which were first introduced by Robert C. Martin (aka “Uncle Bob”) in his book “Agile Software Development: Principles, Patterns, and Practices”.
The SOLID principles are a set of five guiding principles that help software designers create code that is easy to maintain, extend, and evolve over time. They are:
Single Responsibility Principle (SRP): A class should have only one reason to change. This means that a class should have a single, well-defined responsibility and should not be responsible for more than one thing.
Open/Closed Principle (OCP): A class should be open for extension but closed for modification. This means that a class should be designed in such a way that new functionality can be added to it without having to modify the existing code.
Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types. This means that any class that extends or implements another class should be able to be used in place of the base class without breaking the application.
Interface Segregation Principle (ISP): Clients should not be forced to depend upon interfaces that they do not use. This means that a class should have many small, focused interfaces rather than a single, monolithic interface that contains everything.
Dependency Inversion Principle (DIP): High-level modules should not depend upon low-level modules. Both should depend upon abstractions. This means that the relationships between classes should be based on abstractions rather than concrete implementations, so that changes in the low-level classes do not affect the high-level classes.
By following the SOLID principles, software designers can create code that is more modular, extensible, and maintainable. This can help reduce the complexity of the codebase and make it easier to add new features and fix bugs over time.