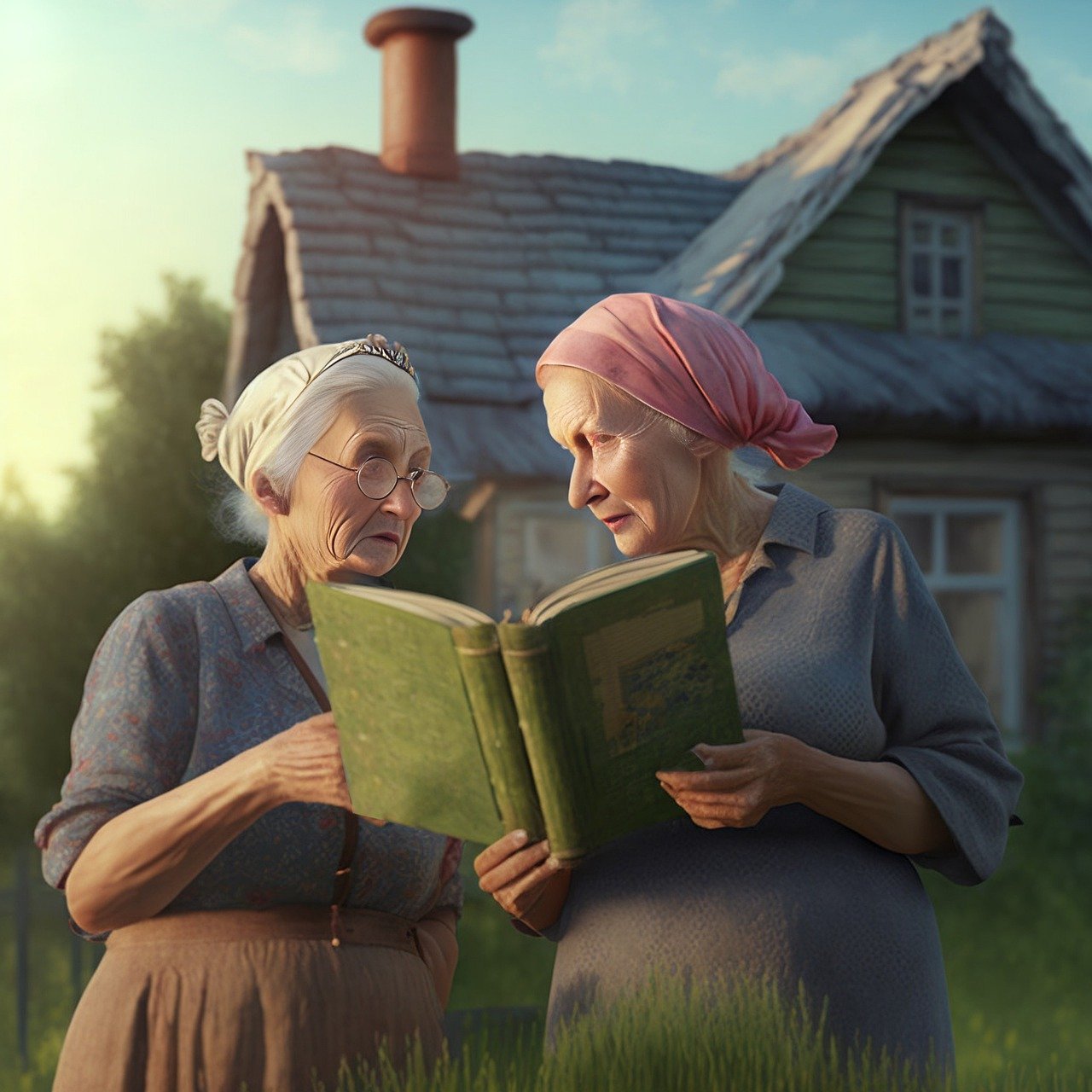
Quick Sort Vs. Merge Sort
Both quick sort and merge sort are efficient sorting algorithms with an average time complexity of O(n log n). However, there are a few differences between the two algorithms that may make one more suitable for a particular situation than the other.
One key difference between quick sort and merge sort is the way they handle sorting large arrays. Quick sort has a worse case time complexity of O(n^2) if the pivot element is not chosen carefully, which can lead to slower performance when sorting large arrays. Merge sort, on the other hand, always has a time complexity of O(n log n) regardless of the input data, making it a more stable and reliable choice for sorting large arrays.
Another difference between the two algorithms is the way they use memory. Quick sort has a relatively small memory footprint as it sorts the array in place, without the need for additional storage. Merge sort, on the other hand, requires additional memory to store the merged arrays, making it less efficient in terms of memory usage.
Here is an example of how to implement quick sort in JavaScript:
function quickSort(arr) {
if (arr.length <= 1) {
return arr;
}
const pivot = arr[0];
const left = [];
const right = [];
for (let i = 1; i < arr.length; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
const sortedArray = quickSort([5, 3, 8, 1, 2, 9, 4]);
console.log(sortedArray); // [1, 2, 3, 4, 5, 8, 9]
And here is an example of how to implement merge sort in JavaScript:
function mergeSort(arr) {
if (arr.length <= 1) {
return arr;
}
const mid = Math.floor(arr.length / 2);
const left = arr.slice(0, mid);
const right = arr.slice(mid);
return merge(mergeSort(left), mergeSort(right));
}
function merge(left, right) {
const result = [];
while (left.length && right.length) {
if (left[0] < right[0]) {
result.push(left.shift());
} else {
result.push(right.shift());
}
}
return [...result, ...left, ...right];
}
const sortedArray = mergeSort([5, 3, 8, 1, 2, 9, 4]);
console.log(sortedArray); // [1, 2, 3, 4, 5, 8, 9]
Ultimately, whether quick sort or merge sort is a better choice will depend on the specific requirements of your application. If you need to sort large arrays with a stable and reliable algorithm, merge sort may be a better choice. If you are working with smaller arrays and need a more efficient use of memory, quick sort may be a better fit. It’s always a good idea to consider the trade-offs between different algorithms and choose the one that best meets your needs.