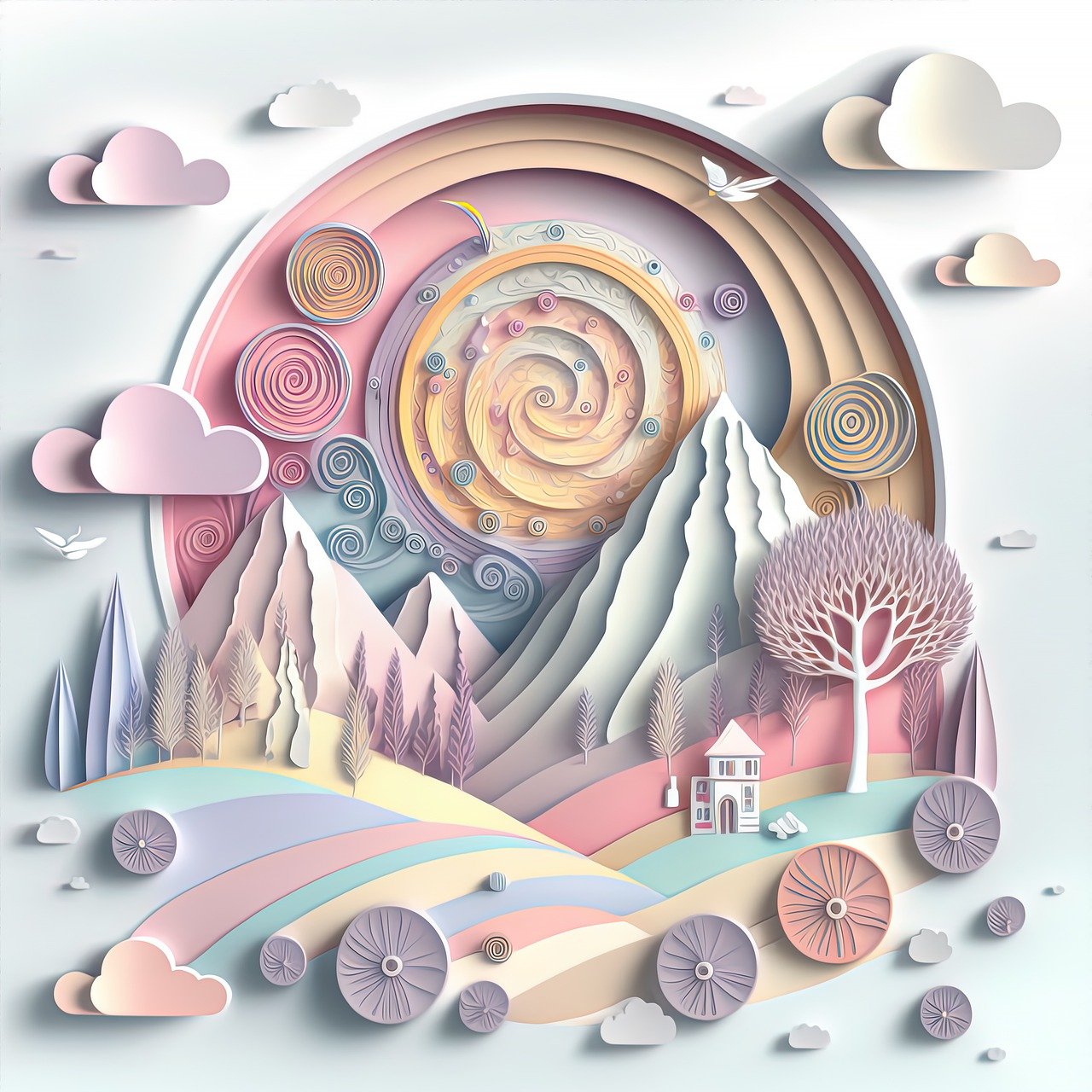
Object oriented programming – Inheritance vs. Composition
In object-oriented programming, inheritance and composition are two different approaches to reusing code and creating relationships between objects.
Inheritance is a mechanism that allows one object to inherit the properties and behavior of another object.
In JavaScript, this is achieved using the extends keyword. For example:
class Animal {
constructor(name) {
this.name = name;
}
makeNoise() {
console.log(`${this.name} is making a noise.`);
}
}
class Dog extends Animal {
constructor(name) {
super(name);
}
bark() {
console.log(`${this.name} is barking.`);
}
}
const dog = new Dog(‘Fido’);
dog.makeNoise(); // Output: “Fido is making a noise.”
dog.bark(); // Output: “Fido is barking.”
In this example, the Dog class extends the Animal class and inherits its makeNoise method. This means that any instance of the Dog class will have access to the makeNoise method, as well as any other methods or properties defined in the Animal class.
Composition, on the other hand, involves creating a new object that contains one or more other objects, and using those objects to implement the desired behavior. In JavaScript, this is typically achieved by using the Object.assign method or the spread operator to combine the properties and methods of multiple objects into a new object.
Here is an example of composition in JavaScript:
const animal = {
makeNoise() {
console.log(‘An animal is making a noise.’);
}
};
const barker = {
bark() {
console.log(‘A barker is barking.’);
}
};
const dog = Object.assign({}, animal, barker);
dog.makeNoise(); // Output: “An animal is making a noise.”
dog.bark(); // Output: “A barker is barking.”
In this example, the dog object is created by combining the animal and barker objects using Object.assign. This gives the dog object access to the methods and properties of both the animal and barker objects.
Both inheritance and composition have their own strengths and weaknesses, and the appropriate approach will depend on the specific needs of your project. Inheritance can be a useful tool for creating relationships between objects, but it can also make it more difficult to change the behavior of an object if the inheritance chain is deep or complex. Composition, on the other hand, can be more flexible and easier to modify, but it can also be more difficult to understand the relationships between objects if the composition is complex.
It is important to carefully consider the trade-offs between inheritance and composition when designing your object-oriented code, and choose the approach that is best suited to your needs.