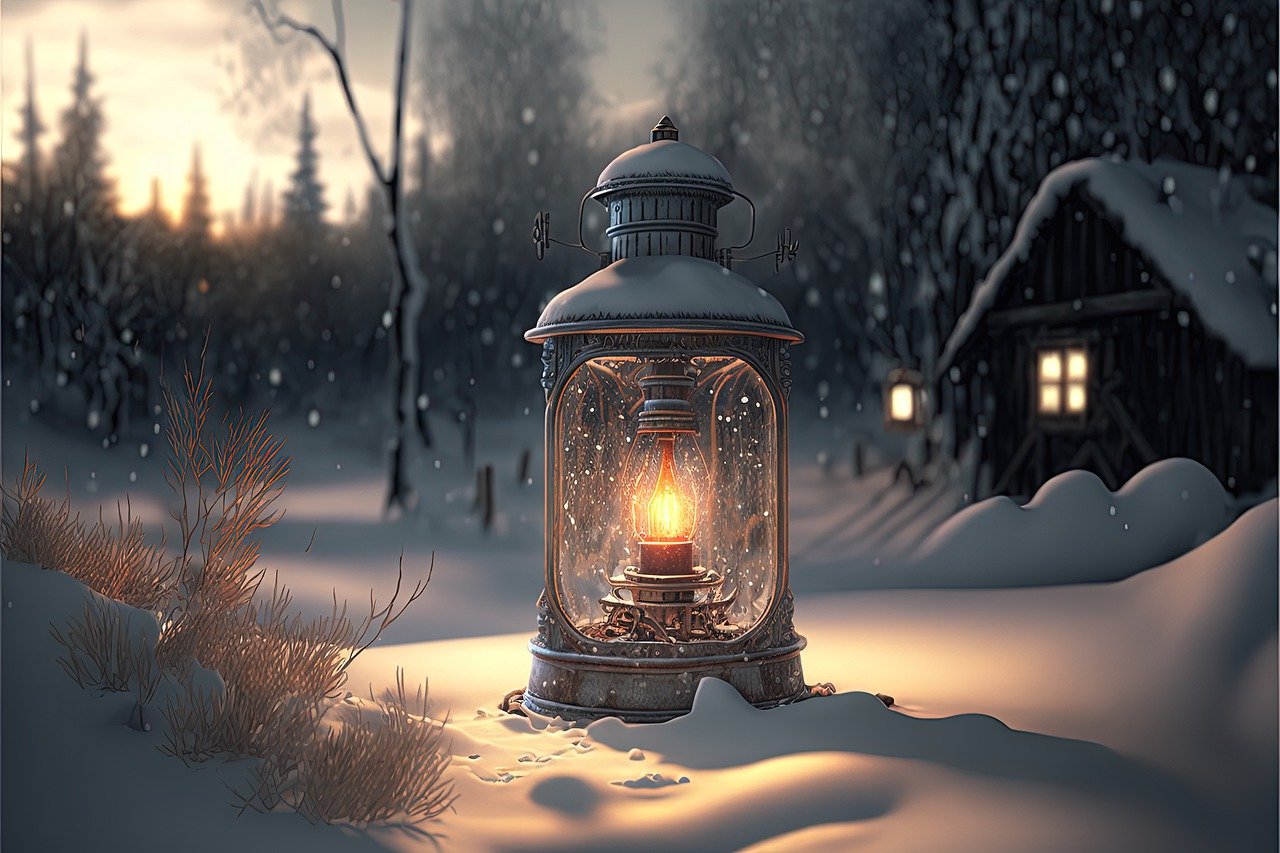
Understanding JavaScript Generators: A Comprehensive Guide with Examples
JavaScript generators are functions that can be paused and resumed multiple times, allowing you to execute code asynchronously and control the flow of execution. In this article, we’ll take a closer look at generators and how they work, and we’ll provide some examples to demonstrate their usage.
What are JavaScript Generators?
JavaScript generators are functions that can be paused and resumed multiple times, allowing you to execute code asynchronously and control the flow of execution. They are denoted by the * symbol before the function keyword, and they use the yield keyword to pause and resume execution.
Here is an example of a simple generator function:
function* generator() {
yield 1;
yield 2;
yield 3;
}
To use a generator function, you need to call it and assign the returned value to a variable. You can then use the next method of the generator object to execute the generator function until the next yield statement is reached. The next method returns an object with two properties: value, which is the value of the yield expression, and done, which is a boolean indicating whether the generator has completed executing.
Here is an example of how you can call a generator function and use the next method to iterate through the generator object:
const generator = generator();
console.log(generator.next().value); // 1
console.log(generator.next().value); // 2
console.log(generator.next().value); // 3
console.log(generator.next().done); // true
You can also use the for...of
loop to iterate through a generator object:
const generator = generator();
for (const value of generator) {
console.log(value);
}
This will output the following:
1
2
3
Why Use JavaScript Generators?
There are several reasons why you might want to use generators in your JavaScript code:
- Asynchronous programming: Generators can be used to execute code asynchronously, allowing you to pause execution and resume it later. This can be particularly useful when working with asynchronous APIs or when performing long-running tasks.
- Control flow: Generators allow you to control the flow of execution, allowing you to pause and resume the generator function at will. This can be useful for debugging or for providing a more intuitive flow of execution. Using generators can allow you to pause and resume the generator function at will, which can be useful for debugging or for providing a more intuitive flow of execution.
JavaScript generators are functions that can be paused and resumed multiple times, allowing you to execute code asynchronously and control the flow of execution. They are denoted by the
*
symbol before the function keyword, and they use theyield
keyword to pause and resume execution. There are several reasons why you might want to use generators in your JavaScript code, including asynchronous programming, control flow, and memory management. In the following section, we’ll provide some examples to demonstrate the use of generators in different contexts.
Copy code
function* generator() {
yield 1;
yield 2;
yield 3;
}
const generator = generator();
for (const value of generator) {
console.log(value);
}