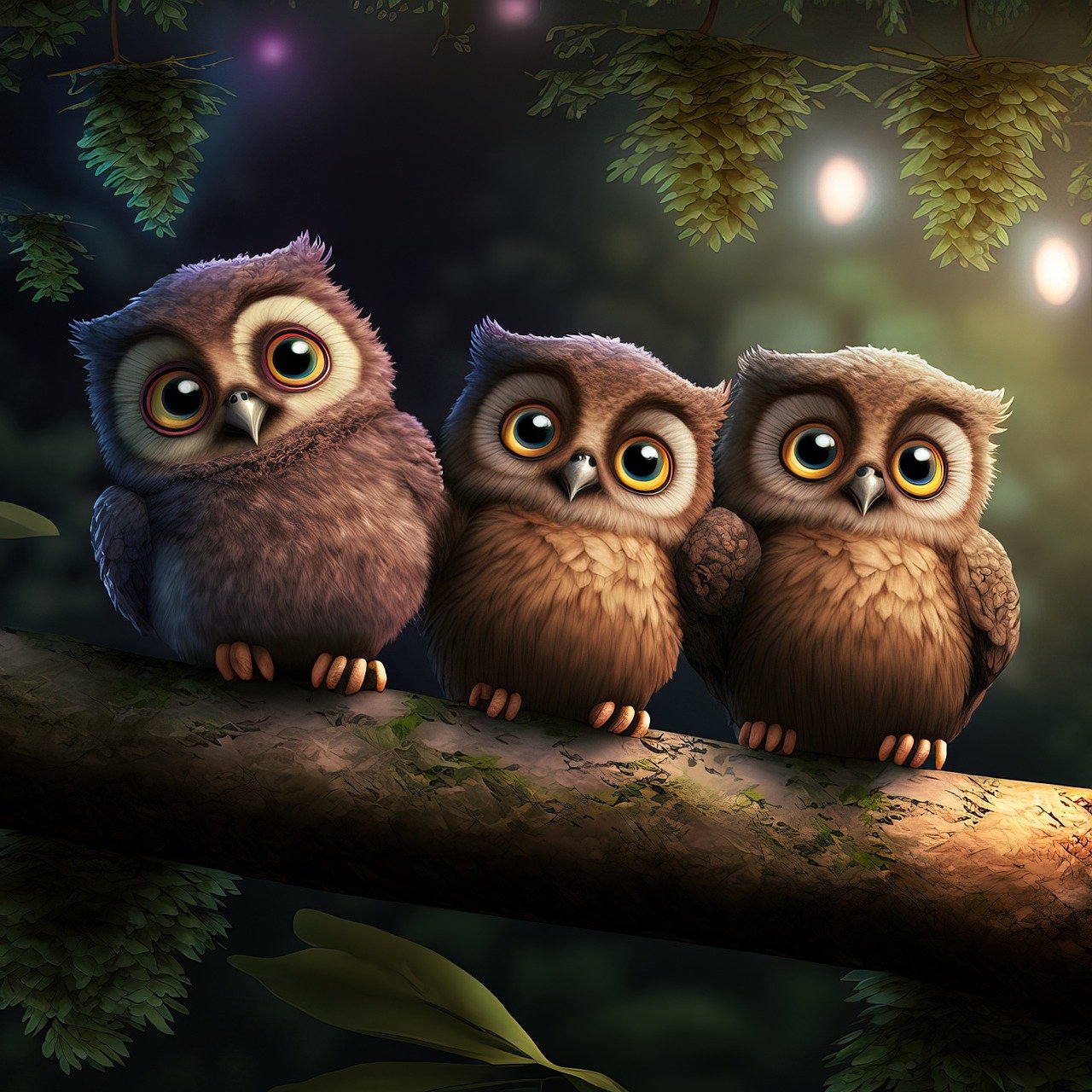
JavaScript immutability
In JavaScript, immutability refers to the idea that once an object or value is created, it cannot be changed.
This can be a useful concept in a number of different contexts, as it allows you to create values that are predictable and easy to understand.
There are a few different ways to achieve immutability in JavaScript. One option is to use the Object.freeze method, which prevents an object from being modified. For example:
const obj = { a: 1, b: 2 };
Object.freeze(obj);
obj.a = 3; // This change will not be allowed
console.log(obj.a); // Output: 1
In this example, the obj object is frozen using the Object.freeze method.
This prevents any changes from being made to the object, so attempting to change the value of obj.a has no effect.
Another option is to use the Object.assign method or the spread operator to create a new object that is a copy of an existing object, rather than modifying the original object.
For example:
const obj = { a: 1, b: 2 };
const newObj = Object.assign({}, obj, { a: 3 });
console.log(obj.a); // Output: 1
console.log(newObj.a); // Output: 3
In this example, the newObj object is created by copying the obj object using Object.assign, and then modifying the value of the a property.
This leaves the original obj object unchanged, while the newObj object has a modified value for the a property.
It is important to note that while these techniques can help to prevent accidental changes to an object or value, they do not completely prevent all forms of mutation.
For example, it is still possible to modify the properties of an object using the delete operator or using the Object.defineProperty method.
However, these techniques can still be useful for creating values that are predictable and easy to understand.
Overall, immutability can be a useful concept in JavaScript, particularly when working with complex data structures or when you want to ensure that values are not changed unexpectedly. By using techniques like Object.freeze or creating new objects rather than modifying existing ones, you can help to create more predictable and maintainable code.