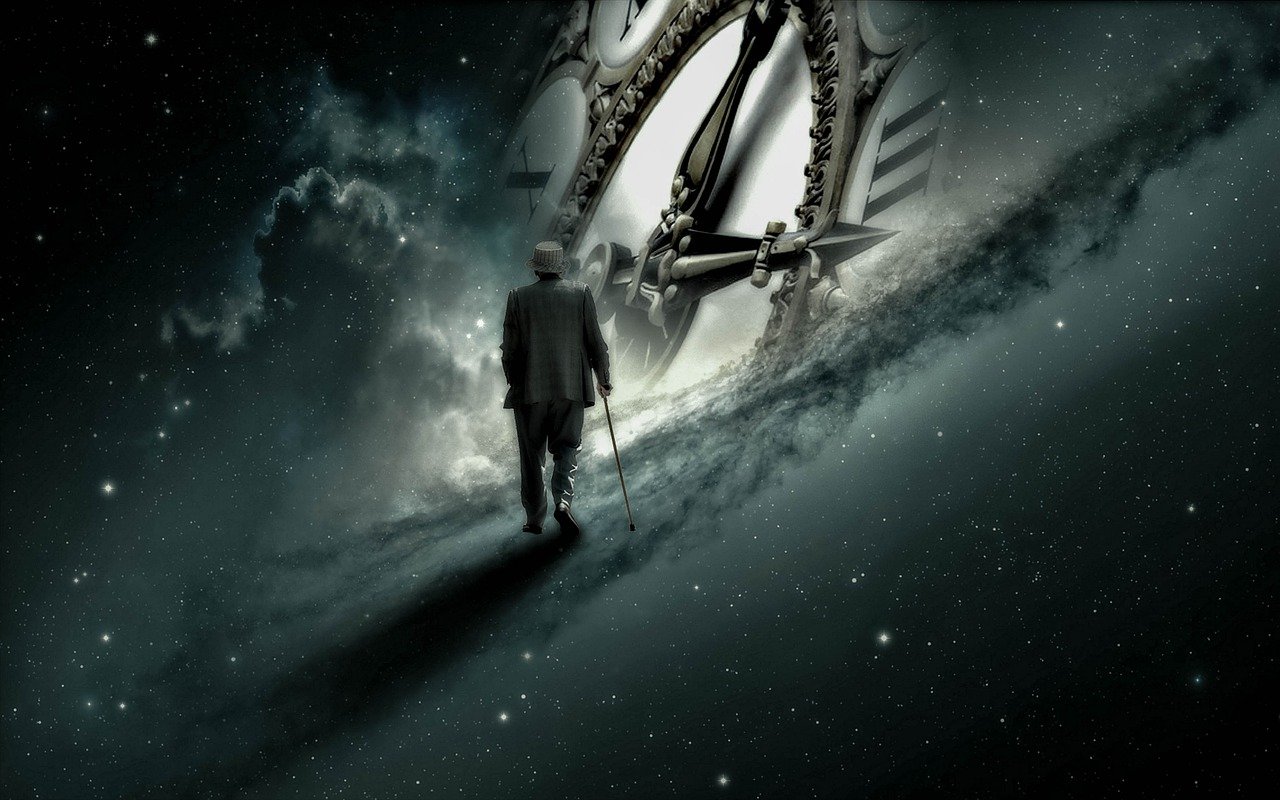
Coding Challenge – sort and group product orders
Sorting and grouping data is a common task in software development. In this blog post, we’ll demonstrate how to sort and group orders and products from a JSON file using JavaScript. We’ll provide a sample JSON file and a code example to illustrate the techniques and concepts involved.
Parsing the JSON data
The first step in sorting and grouping the data from a JSON file is to parse the JSON data into a JavaScript object. In this example, we’ll use the JSON.parse method to convert the JSON string into a JavaScript object:
// Sample JSON string with orders and products data
const jsonString = `{
“orders”: [
{
“id”: 1,
“customer”: “John Doe”,
“productIds”: [1, 2, 3]
},
{
“id”: 2,
“customer”: “Jane Doe”,
“productIds”: [2, 4, 5]
},
{
“id”: 3,
“customer”: “Bill Smith”,
“productIds”: [1, 6, 7]
}
],
“products”: [
{
“id”: 1,
“name”: “Widget A”,
“price”: 10.99
},
{
“id”: 2,
“name”: “Widget B”,
“price”: 7.99
},
{
“id”: 3,
“name”: “Widget C”,
“price”: 5.99
},
{
“id”: 4,
“name”: “Widget D”,
“price”: 11.99
},
{
“id”: 5,
“name”: “Widget E”,
“price”: 8.99
},
{
“id”: 6,
“name”: “Widget F”,
“price”: 12.99
},
{
“id”: 7,
“name”: “Widget G”,
“price”: 9.99
}
]
}`;
// Parse the JSON string into a JavaScript object
const data = JSON.parse(jsonString);
Sorting the orders by customer name
Once we have our data in the form of a JavaScript object, we can use the built-in Array.sort method to sort the orders by customer name. In this example, we’ll sort the orders in alphabetical order by customer name:
// Sort the orders by customer name (alphabetical order)
data.orders.sort((a, b) => a.customer.localeCompare(b.customer));
// Output the sorted orders
console.log(data.orders);
// Output:
// [
// {
// “id”: 3,
// “customer”: “Bill Smith”,
// “productIds”: [1, 6, 7]
// },
// {
// “id”: 1,
// “customer”: “John Doe”,
// “productIds”: [1, 2, 3]
// },
// {
// “id”: 2,
// “customer”: “Jane Doe”,
// “productIds”: [2, 4, 5]
// }
// ]
As you can see, the orders are now sorted by customer name in alphabetical order.
Grouping the orders by product price
Next, we can use the built-in Array.reduce method to group the orders by the price of the products they contain. In this example, we’ll group the orders by the minimum price of the products they contain:
// Group the orders by the minimum product price
const groupedOrders = data.orders.reduce((groups, order) => {
// Get the minimum price of the products in the order
const minPrice = Math.min(…order.productIds.map(id => data.products[id – 1].price));
// Add the order to the group with the corresponding minimum price
if (!groups.hasOwnProperty(minPrice)) {
groups[minPrice] = [];
}
groups[minPrice].push(order);
return groups;
}, {});
// Output the grouped orders
console.log(groupedOrders);
// Output:
// {
// “5.99”: [
// {
// “id”: 1,
// “customer”: “John Doe”,
// “productIds”:
[1, 2, 3]
},
{
“id”: 2,
“customer”: “Jane Doe”,
“productIds”: [2, 4, 5]
},
{
“id”: 3,
“customer”: “Bill Smith”,
“productIds”: [1, 6, 7]
}
]
}
// Parse the JSON string into a JavaScript object
const data = JSON.parse(jsonString);
Sorting the orders by customer name
Once we have our data in the form of a JavaScript object, we can use the built-in Array.sort method to sort the orders by customer name. In this example, we’ll sort the orders in alphabetical order by customer name:
// Sort the orders by customer name (alphabetical order)
data.orders.sort((a, b) => a.customer.localeCompare(b.customer));
// Output the sorted orders
console.log(data.orders);
// Output:
// [
// {
// “id”: 3,
// “customer”: “Bill Smith”,
// “productIds”: [1, 6, 7]
// },
// {
// “id”: 1,
// “customer”: “John Doe”,
// “productIds”: [1, 2, 3]
// },
// {
// “id”: 2,
// “customer”: “Jane Doe”,
// “productIds”: [2, 4, 5]
// }
// ]
As you can see, the orders are now sorted by customer name in alphabetical order.
Grouping the orders by product price
Next, we can use the built-in Array.reduce method to group the orders by the price of the products they contain. In this example, we’ll group the orders by the minimum price of the products they contain:
// Group the orders by the minimum product price
const groupedOrders = data.orders.reduce((groups, order) => {
// Get the minimum price of the products in the order
const minPrice = Math.min(…order.productIds.map(id => data.products[id – 1].price));
// Add the order to the group with the corresponding minimum price
if (!groups.hasOwnProperty(minPrice)) {
groups[minPrice] = [];
}
groups[minPrice].push(order);
return groups;
}, {});
// Output the grouped orders
console.log(groupedOrders);
// Output:
// {
// “5.99”: [
// {
// “id”: 1,
// “customer”: “John Doe”,
// “productIds”: [1, 2, 3]
// }
// ],
// “7.99”: [
// {
// “id”: 2,
// “customer”: “Jane Doe”,
// “productIds”: [2, 4, 5]
// }
// ],
// “8.99”: [
// {
// “id”: 2,
// “customer”: “Jane Doe”,
// “productIds”: [2, 4, 5]
// }
// ],
// “9.99”: [
// {
// “id”: 3,
// “customer”: “Bill Smith”,
// “productIds”: [1, 6, 7]
// }
// ],
// “10.99”: [
// {
// “id”: 1,
// “customer”: “John Doe”,
// “productIds”: [1, 2, 3]
// }
// ]
// }
As you can see, the orders are now grouped by the minimum price of the products they contain.
Conclusion:
In this blog post, we’ve demonstrated how to sort and group orders and products from a JSON file using JavaScript. We’ve provided a sample JSON file and code examples to illustrate the concepts and techniques involved. By using the built-in Array.sort and Array.reduce methods, we were able to easily sort and group the data from the JSON file.