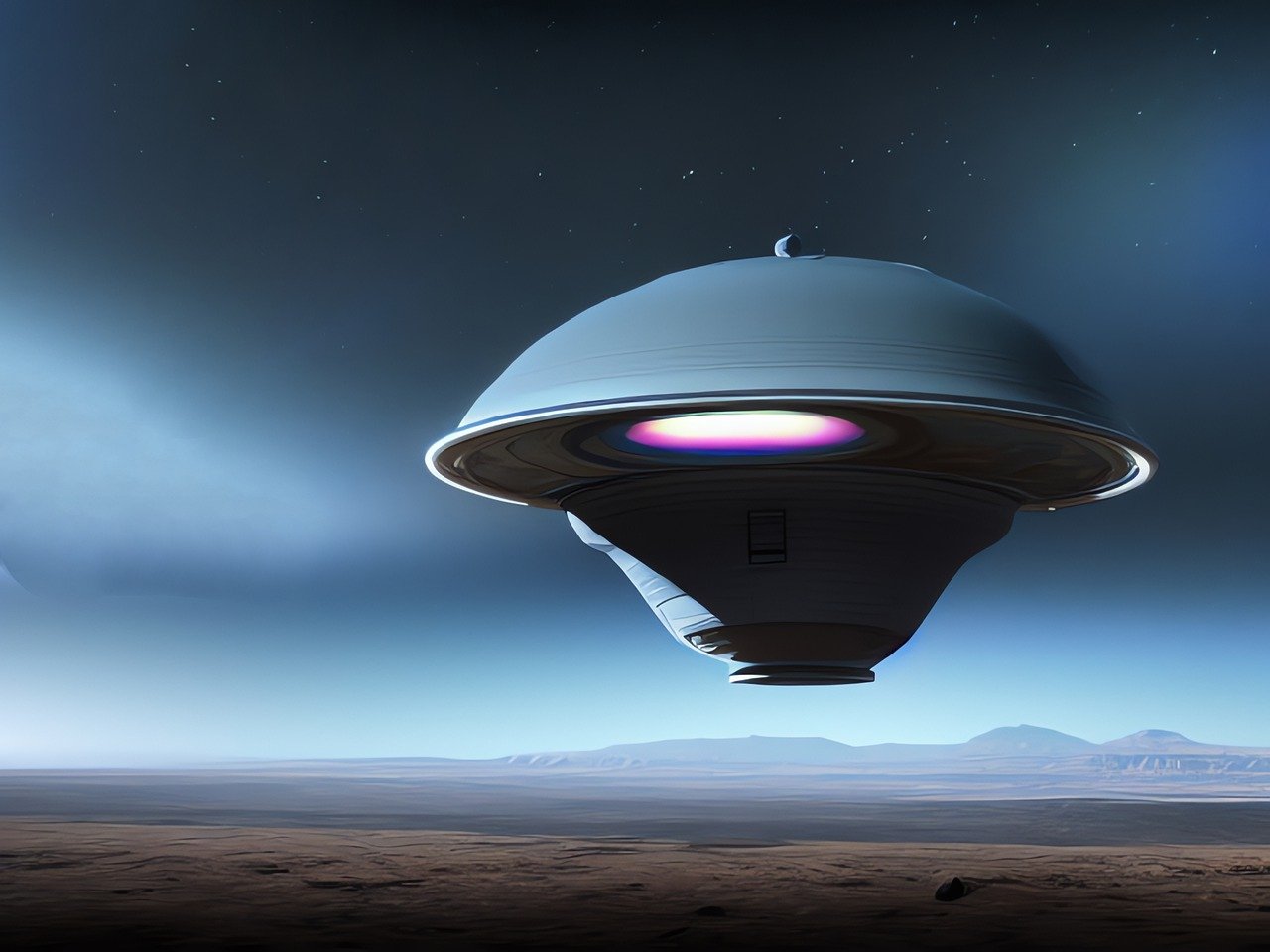
React Render Cycle
Introduction
React is a popular JavaScript library for building user interfaces. It allows developers to create reusable components that can be easily composed to build complex applications. In React, the render cycle is the process by which a component updates its output in response to changes in its state or props.
The Render Cycle
The React render cycle consists of three phases:
- Render: In this phase, React calls the
render
method of a component to compute its output. The output is a description of the component’s UI, written using React elements. - Commit: In this phase, React updates the UI to match the description computed in the render phase. This may involve creating, updating, or destroying React elements and their corresponding DOM nodes.
- Commit Lifecycle: In this phase, React calls the lifecycle methods of a component, such as
componentDidMount
,componentDidUpdate
, andcomponentWillUnmount
, to allow the component to perform side effects, such as fetching data or updating the local state.
Optimizing the Render Cycle
The React render cycle can have a significant impact on the performance of your application. If a component re-renders too often, it can cause the UI to become slow and janky. To optimize the render cycle, you can use the following techniques:
- Use
shouldComponentUpdate
to prevent unnecessary re-renders. - Use
React.PureComponent
The Render Cycle Steps
The React render cycle consists of the following steps:
-
Receiving props and state: When a component is rendered, it receives props and state from its parent. These values are used to initialize the component’s internal state and determine its initial render output.
-
Updating state: If the component’s state or props change, the component will update its internal state and schedule a new render.
-
Should component update: Before rendering, the component may call its
shouldComponentUpdate
method to determine whether it should update the UI. If this method returnsfalse
, the render will be skipped and the component will remain unchanged. -
Render: The component will call its
render
method to generate a React element that represents its current state. -
Updating the DOM: React will compare the new React element with the previous one, and apply any necessary changes to the DOM to make the UI match the element.
-
Committing changes: After updating the DOM, the component will call its
componentDidUpdate
method, which allows it to perform any necessary post-update actions, such as making network