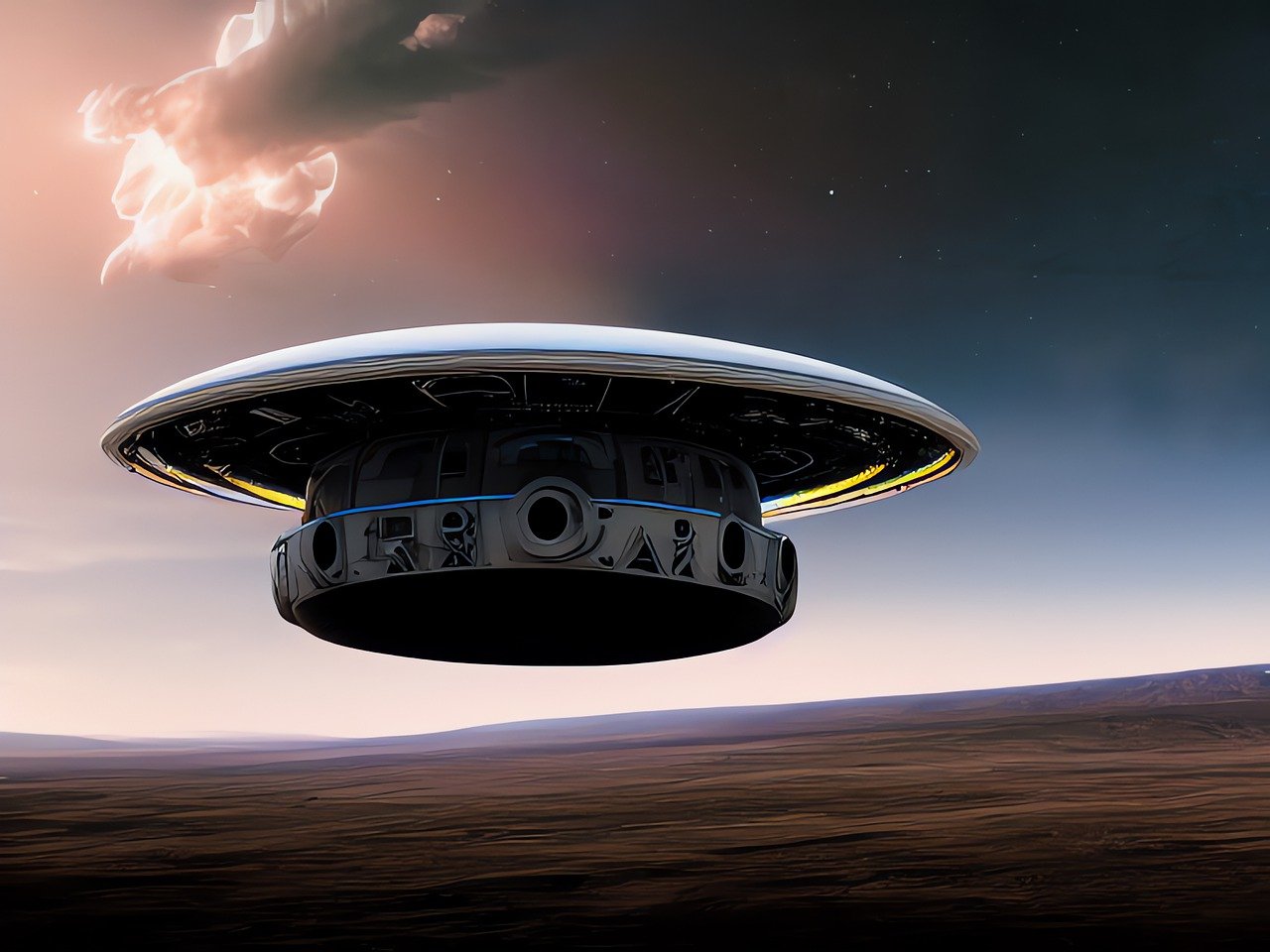
React lifecycle hooks in functional components
Introduction
In React, components can be defined as functions or classes. Function components, also known as stateless components, are components that are defined as JavaScript functions. They do not have a state or lifecycle methods, but they can still be customized using special functions called life cycle hooks.
Life Cycle Hooks
Life cycle hooks are functions that are called at specific points in a component’s life cycle. They allow you to perform actions at those points, such as updating the component’s state or making network requests.
Functional components in React have the following life cycle hooks:
useEffect
: This hook is called after the component is rendered and after every update. It allows you to perform side effects, such as making network requests or updating the component’s state.useLayoutEffect
: This hook is similar touseEffect
, but it is called synchronously after the component is rendered and after every update. This allows you to perform layout updates, such as measuring the size of an element.useMemo
: This hook is called before every update, and it allows you to memoize a value. This means that the value will be computed and cached, and it will only be recomputed if one of its dependencies changes.useCallback
: This hook is similar touseMemo
, but it is used to memoize a function. This allows you to avoid creating a new function on every render, which can improve performance.
Here is an example of a functional component using life cycle hooks:
import * as React from "react";
const MyComponent = (props) => {
const [count, setCount] = React.useState(0);
const increment = React.useCallback(() => setCount(count + 1), [count]);
React.useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
export default MyComponent;
In the example above, the MyComponent
component uses the useState
hook to initialize and update its internal state. It also uses the useCallback
hook to memoize the increment
function, which is used to increment the count. Finally, it uses the useEffect
hook to update the document title whenever the count changes.
Conclusion
Functional components in React have life cycle hooks that allow you to perform actions at specific points in a component’s life cycle. These hooks can be used to perform side effects, memoize values, and update the component’s state and UI.