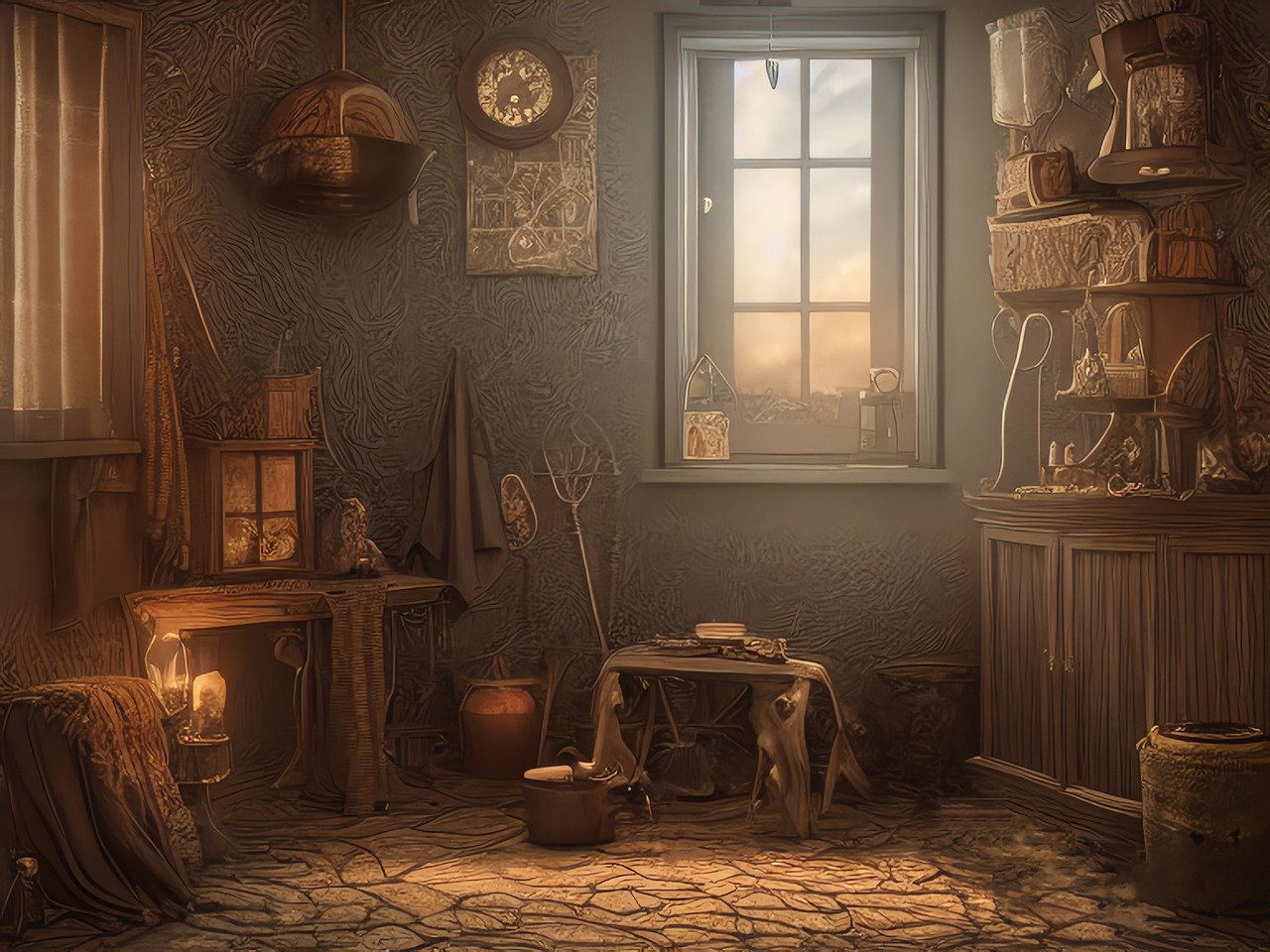
Using TypeScript with React
Introduction
React is a popular JavaScript library for building user interfaces. It allows developers to create reusable components that can be easily composed to build complex applications. TypeScript is a programming language that is a superset of JavaScript. It adds optional static typing and other features to JavaScript, allowing developers to write type-safe code and catch errors at compile time.
Using TypeScript with React
TypeScript can be used with React to add type-safety to your code and catch errors at compile time. To use TypeScript with React, you will need to install the typescript
and @types/react
packages. You can do this using the following command:
npm install typescript
/reactOnce you have installed these packages, you can create a TypeScript file for your React component. For example, if you have a component named MyComponent
, you can create a file named MyComponent.tsx
for it. The .tsx
file extension indicates that the file contains TypeScript and JSX code.
Here is an example of a simple React component written in TypeScript:
import * as React from "react";
interface Props {
name: string;
}
const MyComponent: React.FC<Props> = (props) => {
return <div>Hello, {props.name}!</div>;
};
export default MyComponent;
In the example above, the component is defined as a function component using the React.FC
generic type. The Props
interface defines the type for the component’s props. The component’s props are then typed using the Props
interface in the MyComponent
function.
Conclusion
Using TypeScript with React allows you to add type-safety to your code and catch errors at compile time. This can help you write safer and more accurate code, and make it easier to refactor and maintain your React components.